When you have products with no SKU and you would like to set Incremental SKU numbers for them directly in the website – then this snippet will help you.
<?phpif(isset($_GET["set_sku"]))add_action('wp',function(){ $base_sku = 100; $args = array( 'post_type' => 'product', 'post_status' => 'publish', 'meta_query' => array( array( 'key' => '_sku', 'compare' => 'NOT EXISTS' ) ) ); $query = new WP_Query($args); while ($query->have_posts()) { $query->the_post(); $product_id = get_the_ID(); $sku = $base_sku++; update_post_meta($product_id, '_sku', "SK_".$sku); } wp_reset_query(); die("DONE. You can remove this code from your website!");});
That’s it. Now just run yourwebsitecom/?set_sku=1 once and done!
The code will create SK_100, SK_101, … SKU-s for your website.
If you want to create SKU-s for variation as well, we can improve our code and get this snippet below:
<?php
if(isset($_GET["set_sku_variations"]))
add_action('wp',function(){
$args = array(
'post_type' => 'product_variation',
'post_status' => 'publish',
'meta_query' => array(
array(
'key' => '_sku',
'compare' => 'NOT EXISTS'
)
)
);
$query = new WP_Query($args);
$base_sku = [];
while ($query->have_posts()) {
$query->the_post();
$product_id = get_the_ID();
$product_parent=get_post_parent();
if(empty($base_sku[$product_parent]))$base_sku[$product_parent]=1;
$sku = $base_sku[$product_parent];
update_post_meta($product_id, '_sku', "SK_".$product_parent."_".$sku);
$base_sku[$product_parent]++;
}
wp_reset_query();
die("DONE. You can remove this code from your website!");
});
This will create incremental variation SKUs for each product. Done with Incremental SKU numbers.
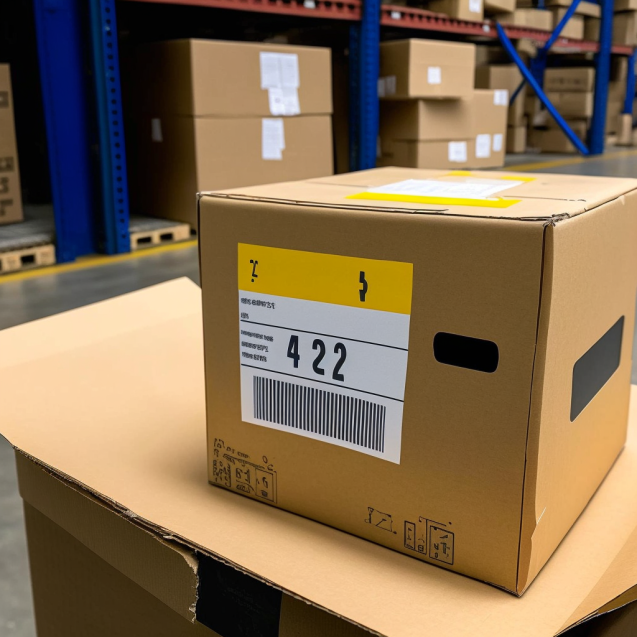
F.e. if your product with ID=877 has 5 children. Then their children will have the SKUs like SK_877_1, SK_877_2, SK_877_3, SK_877_4 and SK_877_5.
For more WooCommerce snippets, check the WooCommerce tag page.